So I wanted to look up a Big Bang Theory episode yesterday and I noticed that Google found quite a number of pages referencing it. As a result, I got curious how many results each of the episodes would return. A few lines of Perl code later, here is the result.
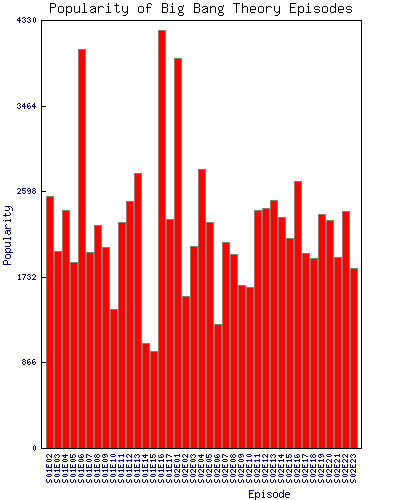
It lists the number of results returned for each episode as a measure of relative popularity. Interesting… By the way, I had to cut out the pilot episode because it turns out that the NSTA pilot’s certification requires you to know about the Big Bang Theory… Huh, who knew!? ;]
Here is the Perl code for the previous Big Bang Theory chart. In case you’re interested… ;]
Edited 2011-11-12 to improve the Perl code, removed dependency on having video files to pull titles from and pull from the Wikipedia Big Bang Episodes list article instead.
#!/usr/bin/env perl
use strict;
use warnings;
use LWP;
use Data::Dumper;
use File::Find;
use GD::Graph::bars;
use GD::Graph::colour qw(:colours :lists :files :convert);
use List::Util qw /max/;
my $googleAPIkey = 'INSERT-YOUR-KEY-HERE';
my $googurl = "http://ajax.googleapis.com/ajax/services/search/web?v=1.0&q=";
my %results = ();
my @csv;
my @episodes = &getEpisodeList();
foreach (@episodes) {
if (!($_ =~ /pilot/i)) {
$results{$_} = dosearch($_);
push(@csv, [$_, $_, $results{$_}]);
sleep 10; # Sleep for a while to avoid triggering quota issues
}
}
my @values;
my @keys = sort(keys(%results));
my $key;
foreach $key (sort(keys(%results))) {
push(@values, $results{$key});
}
my $maxYVal = (max @values) + 100;
print $maxYVal;
my $graph = GD::Graph::bars->new(800,600);
$graph->set(
x_label => 'Episode',
y_label => 'Popularity',
title => 'Popularity of Big Bang Theory Episodes',
y_max_value => $maxYVal,
transparent => 0,
bgclr => qw(white),
fgclr => qw(black),
textclr => qw(black),
x_labels_vertical => 1
) or die $graph->error;
my @data = (
[@keys],
[@values]
);
my $gd = $graph->plot(\@data) or die $graph->error;
open(IMG, '>BigBangTheoryPopularityChart.png') or die $!;
binmode IMG;
print IMG $gd->png;
close IMG;
open CSV, ">BigBangTheoryGraphData.csv";
foreach (@csv) {
print CSV join(",", @$_), "\n";
}
sub getEpisodeList() {
my @episodes = `curl http://en.wikipedia.org/wiki/List_of_The_Big_Bang_Theory_episodes | grep '\\([a-zA-Z0-9 ]*\\).*/\\1/' -e 's/]*>//g' -e 's/"//g'`;
return @episodes;
}
sub dosearch {
chomp @_; my ($term) = @_;
$term =~ s/ /+/g;
my $agent = LWP::UserAgent->new();
my $search = "${googurl}The+Big+Bang+Theory+${term}&key=${googleAPIkey}";
$agent->timeout(1800);
print "$search\n";
my $response = $agent->get($search);
die "Cant't get $googurl -- ", $response->status_line unless $response->is_success;
my $resp_content = $response->content;
# print "$resp_content\n";
if ($resp_content =~ /"estimatedResultCount":"(\d+)"/) {
print "$1 results\n";
return $1;
} else {
return 0;
}
}